Memory management is a fundamental aspect of programming, essential to the stability and performance of applications. Among the challenges associated with managing memory is the phenomenon of memory leaks, which can significantly degrade the performance of an application or even cause it to crash. This article delves into what memory leaks are, their causes, how they can be detected, and methods to prevent them. Additionally, it includes practical coding examples and discusses how the use of SMART TS XL can enhance the detection, analysis, and prevention of memory leaks through advanced static analysis, flowchart building, and code quality improvements.
What Are Memory Leaks?
A memory leak occurs when a program allocates memory from the heap but fails to release it back when it’s no longer needed. As a result, the memory is no longer in use by the program but cannot be reclaimed by the operating system or other processes. Over time, these unreleased blocks of memory accumulate, reducing the amount of available memory, which can lead to reduced performance and eventually to the program crashing if the system runs out of memory.
In managed languages like Java or C#, memory management is handled by the garbage collector, which automatically reclaims memory that is no longer referenced. However, even in these environments, memory leaks can occur if objects are still referenced inadvertently, preventing the garbage collector from freeing the memory.
Causes of Memory Leaks
Memory leaks can arise from a variety of situations, often depending on the programming language and environment in use. Here are some common causes:
Unreleased Resources
When a program uses resources such as file handles, database connections, or GUI objects, these resources need to be explicitly released after use. Failure to do so keeps the memory associated with these resources allocated, leading to a memory leak.
Circular References
In languages like Python, which use reference counting for memory management, circular references can cause memory leaks. This happens when two or more objects reference each other, creating a cycle that the garbage collector cannot resolve, leading to memory not being freed.
Failure to Deallocate Memory
In languages that require manual memory management, such as C or C++, forgetting to free memory that was dynamically allocated results in memory leaks. This is often due to programming errors where the developer forgets to include the necessary free() or delete calls after the memory is no longer needed.
Listener or Observer Patterns
In event-driven programming, listeners or observers are used to handle events or changes in state. If these listeners are not properly deregistered, they continue to reference the objects, preventing the memory from being released, even when the objects are no longer in use.
Caching without Expiry
Implementing a cache can introduce memory leaks if there’s no proper expiration or removal mechanism for old or unused items. Over time, the cache grows and consumes more memory, even if the cached data is no longer needed.
Global Variables
Using global variables can lead to memory leaks if the data stored in them is not properly managed. Since global variables persist for the lifetime of the program, any memory they hold will not be freed until the program exits.
Improper Use of Third-Party Libraries
Sometimes memory leaks can occur due to bugs or improper usage of third-party libraries, where the library does not correctly manage memory, or the developer does not properly integrate the library with the application’s memory management strategy.
Detection of Memory Leaks
Detecting memory leaks is essential for maintaining the health of an application. Various tools and techniques can be employed to identify and address memory leaks:
Static Code Analysis
Traditional static code analysis tools are often used to identify potential memory leaks by analyzing the source code without running the program. SMART TS XL can be a superior solution, offering enhanced static analysis capabilities, which can more accurately detect patterns in the code that may lead to memory management issues, such as unfreed memory allocations or unreleased resources.
Heap Profiling
Heap profilers like Valgrind (for C/C++), VisualVM (for Java), and .NET Memory Profiler monitor memory usage during program execution. They help identify which parts of the program are consuming the most memory and whether any objects are persisting longer than expected. SMART TS XL can be integrated with these profiling tools to provide more detailed and actionable insights into memory usage and potential leaks.
Flowchart Building
SMART TS XL can automatically generate flowcharts from your code, helping visualize the memory allocation and deallocation processes. This graphical representation makes it easier to spot areas where memory leaks might occur, especially in complex codebases where tracking memory management manually is challenging.
Memory Leak Detection Tools
Specialized tools like AddressSanitizer, Dr. Memory, and LeakSanitizer are designed to detect memory leaks at runtime. Integrating these with SMART TS XL allows for the results to be cross-referenced with static analysis findings, providing a more comprehensive understanding of where and why memory leaks occur.
Manual Code Review
Manual code reviews by experienced developers can sometimes catch memory leaks that automated tools might miss. SMART TS XL can assist in this process by highlighting suspicious code patterns and areas that are prone to memory leaks, thus streamlining the code review process.
Impact Analysis
SMART TS XL can perform impact analysis to determine how changes in one part of the code may affect memory management in other areas. This is particularly useful in large projects where even small changes can have far-reaching consequences on memory usage and potential leaks.
Prevention of Memory Leaks
Preventing memory leaks is often more efficient than trying to fix them after the fact. Here are some strategies to prevent memory leaks during the development process:
Use RAII (Resource Acquisition Is Initialization)
In C++, RAII is a common idiom where resources are tied to the lifetime of an object. When an object goes out of scope, its destructor automatically releases the associated resources. This pattern ensures that memory is freed and resources are released when they are no longer needed.
Smart Pointers
In languages like C++, smart pointers such as std::unique_ptr and std::shared_ptr automate memory management. They automatically free memory when it’s no longer in use, reducing the risk of memory leaks. However, developers must still be cautious about circular references when using std::shared_ptr.
Avoid Global Variables
Limiting the use of global variables can reduce the likelihood of memory leaks. When global variables are necessary, it’s important to carefully manage the memory they use and ensure it’s released appropriately.
Proper Resource Management
Always ensure that resources like file handles, sockets, and database connections are properly closed or released after use. This can be achieved through the use of try-finally blocks in languages like Java and Python, or by using constructs like the using statement in C# that automatically handles resource cleanup.
Weak References
In languages like Java, where strong references prevent garbage collection, weak references can be used to avoid memory leaks. Weak references do not prevent their referents from being collected by the garbage collector, thus allowing the memory to be reclaimed when it’s no longer in use.
Implement Caching Strategies
If using caches, implement strategies like Least Recently Used (LRU) eviction, time-to-live (TTL) policies, or reference counting to ensure that old or unused cache entries are removed and do not cause memory leaks.
Unit Testing for Memory Leaks
Incorporating memory leak detection into unit tests can help catch leaks early in the development cycle. Tools like Valgrind can be integrated into the testing process to ensure that new code does not introduce memory leaks.
Code Quality Improvement
SMART TS XL goes beyond static analysis by providing actionable insights to improve overall code quality. By identifying potential memory management issues early, developers can write more robust and leak-resistant code. The tool’s impact analysis and integration with other development tools make it a powerful asset in preventing memory leaks before they occur.
Memory Leaks Case Studies and Examples
To better understand the impact of memory leaks and their resolution, let’s consider a few real-world examples:
Mozilla Firefox (2006)
Firefox was once notorious for its memory leaks, especially in earlier versions. The problem was exacerbated by the fact that many users kept their browsers open for extended periods. The leaks were primarily due to cycles in the document object model (DOM) and unintentional retention of JavaScript objects. Mozilla addressed these issues through significant efforts to refactor code and introduce better memory management practices, leading to improved stability and performance in later versions.
JavaScript Single-Page Applications (SPAs)
SPAs often suffer from memory leaks due to the dynamic nature of content creation and destruction. For example, if event listeners are not properly removed when elements are deleted, the memory associated with those elements cannot be reclaimed. Frameworks like React and Angular have introduced mechanisms to help manage such issues, but developers still need to be vigilant in ensuring that components are correctly disposed of.
Mobile Applications
Mobile apps, particularly on Android, can be prone to memory leaks due to the constrained environment. For instance, activities in Android can cause leaks if they hold references to long-lived objects like singletons or static variables. Developers often use tools like LeakCanary to monitor and fix memory leaks in mobile applications, ensuring smoother performance and preventing crashes.
Coding Samples
Here are some coding examples that demonstrate common memory leaks and their resolutions:
C++ Example: Manual Memory Management
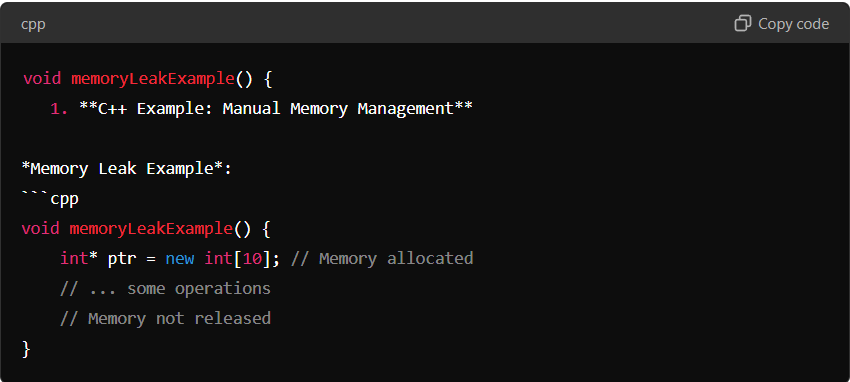
In this example, memory is allocated using new[] to create an array of integers. However, the memory is not released because there is no delete[] call to free it, leading to a memory leak.
Resolved Example:
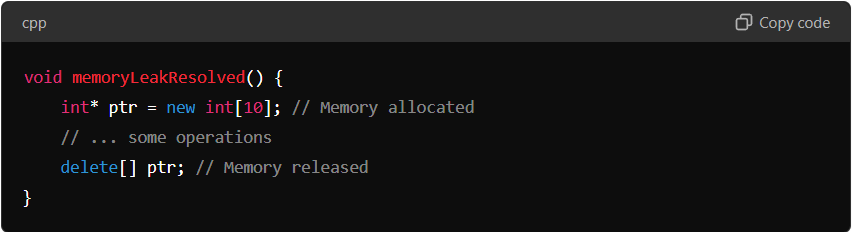
To resolve the leak, the allocated memory is properly freed using delete[]. This ensures that the memory is returned to the system once it’s no longer needed.
Java Example: Listener Memory Leak
Memory Leak Example:
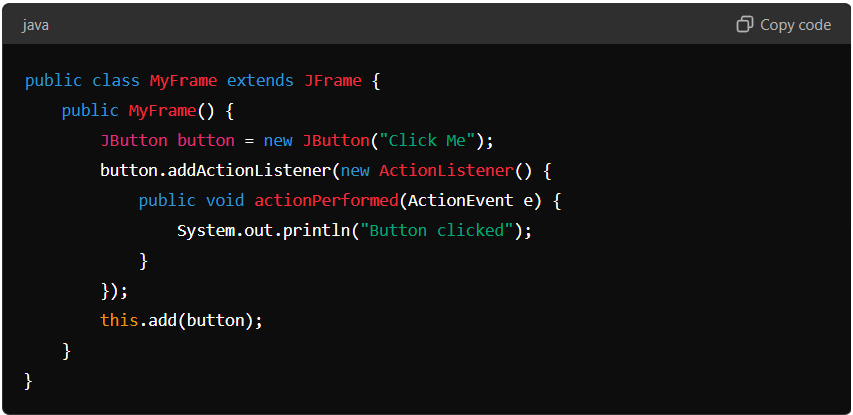
In this example, an anonymous inner class is used to create an ActionListener for a button. However, if the button is removed or the frame is closed without removing the listener, the listener may cause a memory leak by keeping the button or frame in memory.
Resolved Example:
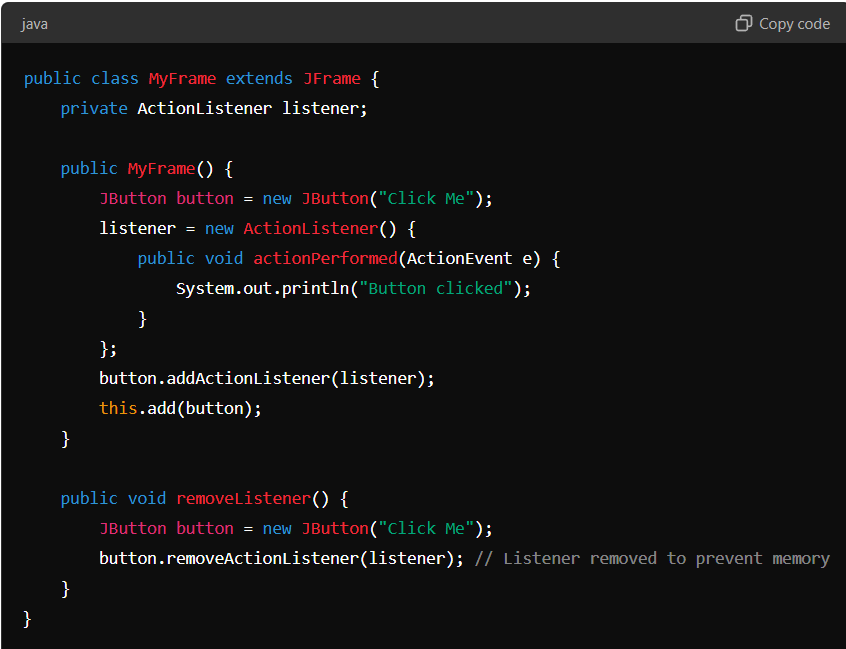
By keeping a reference to the listener and explicitly removing it when the button is no longer needed, the potential for a memory leak is mitigated.
Python Example: Circular Reference
Memory Leak Example:
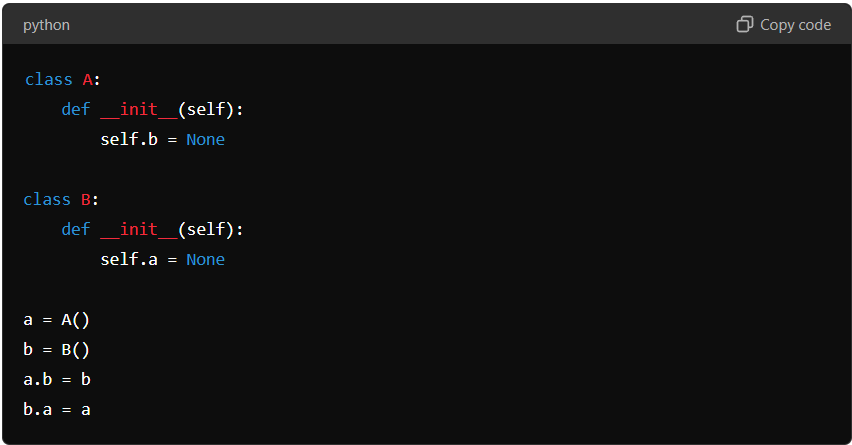
In this example, a and b hold references to each other, creating a circular reference. This can prevent Python’s garbage collector from freeing the objects, causing a memory leak.
Resolved Example:
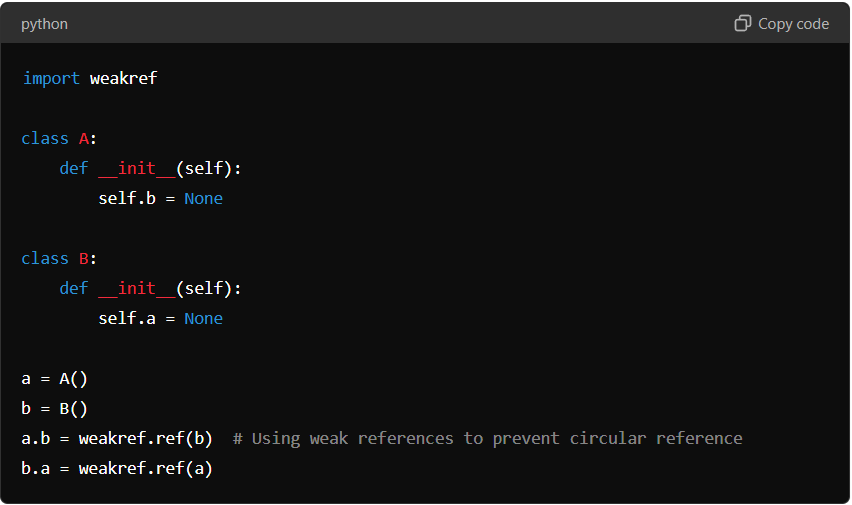
By using weakref, the circular reference is broken, allowing the garbage collector to reclaim the memory when the objects are no longer in use.
SMART TS XL: A Tool for Effective Memory Leak Detection and Resolution
SMART TS XL can significantly enhance the process of detecting and resolving memory leaks. Here’s how this tool can be integrated into your development workflow:
Static Code Analysis: SMART TS XL offers advanced static analysis capabilities, identifying potential memory leaks by analyzing your code. Unlike other tools, it provides deeper insights and more accurate detection of patterns that can lead to memory leaks.
Flowchart Building: SMART TS XL can automatically generate flowcharts that visualize the memory allocation and deallocation processes within your code. This feature is particularly useful for understanding complex memory management scenarios and identifying where leaks might occur.
Impact Analysis: With SMART TS XL, you can perform impact analysis to see how changes in one part of the code might affect memory management in other areas. This is especially beneficial in large projects where even minor changes can have significant repercussions on memory usage.
Code Quality Improvement: Beyond just detecting leaks, SMART TS XL provides suggestions for improving overall code quality, helping you write more robust, maintainable, and leak-resistant code.
By incorporating SMART TS XL into your development process, you can significantly reduce the risk of memory leaks and ensure that your applications remain stable and efficient. Whether you’re dealing with manual memory management in C++ or handling object references in managed languages like Java and Python, SMART TS XL offers the tools you need to maintain high standards of memory management and overall code quality.